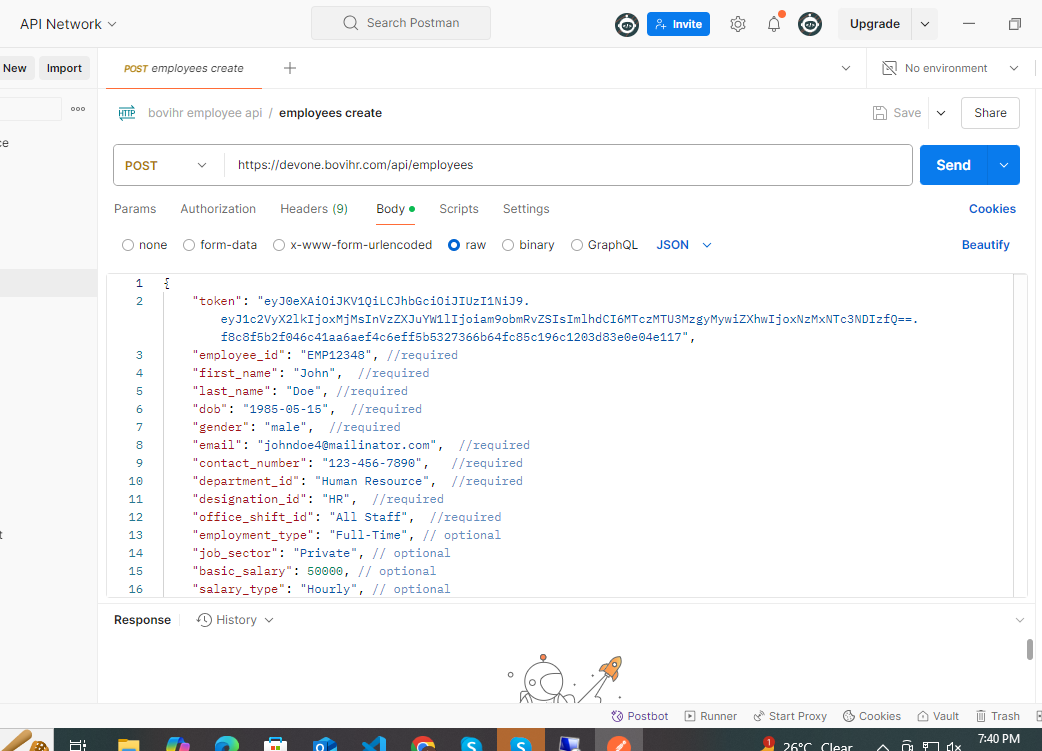
1. Input Validation and Token Verification
- The function starts by validating the incoming data to ensure that required fields are provided, and checks for data consistency such as unique employee ID, email, and username.
- It checks whether the provided token is valid, not expired, and whether the user has the necessary permissions to create an employee.
2. Employee Data Validation
- The employee data (like employee_id, first_name, last_name, dob, gender, etc.) is validated using predefined rules.
- It checks if the employee ID, email, and username are unique, and also validates if related data such as department, designation, shift, and role exist in the system.
3. Mapping and Assigning Data
- Maps various optional fields such as employment_type, job_sector, salary_type, and account_type based on internal mappings.
- It ensures the provided data for national health insurance, social security, TIN, and other optional fields are correctly handled and stored.
4. Database Operations
- User Creation: Creates a user record with details like first_name, last_name, email, username, and password.
- Employee Details: Creates an associated employee record in the staffdetails table, linking the user to their job information (e.g., department_id, designation_id, office_shift_id, salary, etc.).
- Field Updates: If additional information (like health insurance or social security numbers) is provided, it updates or inserts those fields into relevant tables.
5. Bank and Contract Details
- If bank details (account_title, account_number) are provided, they are stored in a bank details table.
- If a contract date is provided, it updates the employee's contract information.
6. Final Response
- If all operations succeed, it returns a success response with a message indicating the employee was created successfully.
Key Features:
- Role and Permission Checking: Ensures that the API user has permission to create employees.
- Unique Checks: Prevents the creation of duplicate employee IDs, emails, and usernames.
- Flexible Fields: Handles both required and optional employee fields, including personal, job-related, and financial information.
- Validation: Strong validation for data integrity, ensuring the data conforms to expected formats.
This function is well-structured for creating an employee, including various checks, validations, and the ability to handle a wide variety of employee-related data, from personal information to financial details.